React hooks
What are hooks in react and how to use them
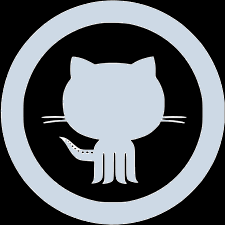
✔️ Hooks are functions that let you "hook into" React state and lifecycle features from function components.
- write function-based components instead of class-based components
- make stateful logic code modular by decoupling it from the class-based component instance and from
this
- make component lifecycle code modular by decoupling it from the class-based component lifecycle methods
✔️ Note : the exact same results could be achieved with classes with clever use of function.prototype.bind
and this
, but it would make the resulting code too verbose and the whole react paradigm less accessible
- function-based components are not classes, so they are hook-compatible to begin with even if they use no hook at all
- hooks must only be called at the top level of react function components
- conditional / iteration logic must be embedded inside the hook (no hook should be called conditionally or iteratively inside a function component)
- the hooks imports are prefixed with "use" because they are executed at each render even if they appear only once in the code
- instead of storing a component's state as properties of
this.state
, the state is now managed on a variable-by-variable basis with theuseState
hook - by construction, the
useState
hook cannot perform partial updates or shallow object merging, only replace the part of the component's state it manages
- effect hooks are created with
useEffect
and will be triggered after the React DOM updates - they can be used to perform network requests, manual DOM mutations, logging ...
- as such, they are similar to component lifecycle post-render events
componentDidMount
andcomponentDidUpdate
- multiple effects hooks can be included in a component and will be executed execute in their declaration order
- effect hooks also accept a "dependency list" parameter :
- if passed an array of values (props, state or whatever) the effect will be triggered after the next render each time any of those values change
- if passed an empty array the effect will be triggered only after the initial render
- if not passed at all, the effect will be triggered after each render
- if an effect hook returns a function, said function will will execute at component unmount (similar to
componentWillUnmount
) - thus this is the place to perform the component teardown / cleanup
- the
useRef
hook returns a mutable object whose.current
property is initialized to the passed argument - the returned object will then persist for the full lifetime of the component and is decoupled from the state / render / effect mechanism
- a notable use case is to create references to the DOM from inside the React DOM and to bind the reference to the actual DOM element at render time