NPM overview
How to configure and use the Node Packages Manager
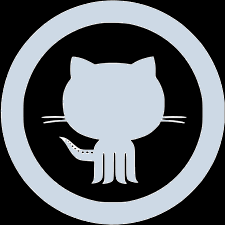
✔️ npm prefix configuration (defaults to where node is installed)
npm config list -l | grep -E "^prefix\ =.*$"
✔️ global install using apt/dpkg
<npm-prefix>/bin # npm executables
<npm-prefix>/share/man # man pages
<npm-prefix>/lib/node_modules # globally installed packages
<npm-prefix>/etc/.npmrc # global config file
$HOME/.npmrc # per-user config file
✔️ global install using nvm
<npm-prefix>/lib/node_modules/npm/bin # npm executables
<npm-prefix>/lib/node_modules/npm/man # man pages
<npm-prefix>/lib/node_modules # globally installed packages
$HOME/.nvm/.npmrc # global config file
$HOME/.npmrc # per-user config file
✔️ local install
<project-path>/node_modules # locally installed npm packages
<project-path>/.npmrc # local config file
Any package present in package.json WILL be locally installed, regardless of it already being globally installed
# prints installed npm version as well as all versions of all binaries/libraries relevant to npm (node, v8, uv, openssl, etc ...)
npm version --versions
# lists all available configuration options
npm config list -l
✔️ when executing a command, config files are read in this order (newer values replace older values in the process)
- npm internal values
- global config file
- per-user config file
- local config file
✔️ list of configs options to override (example)
color=true
description=true
init-license=MIT
init-version=1.0.0
location=user
script-shell=/bin/bash
searchlimit=50
tag=latest
update-notifier=true
userconfig=~/.npmrc
viewer=man
-
When installing locally, npm first tries to find an appropriate prefix folder. This is so that npm install foo@1.2.3 will install to the sensible root of your package, even if you happen to have cded into some other folder.
-
Starting at the $PWD, npm will walk up the folder tree checking for a folder that contains either a package.json file, or a node_modules folder.
-
If such a thing is found, then that is treated as the effective "current directory" for the purpose of running npm commands (this behavior is inspired by and similar to git's .git-folder seeking logic when running git commands in a working dir.)
-
If no package root is found, then the current folder is used.
-
In extreme cases, the configuration parameter values can be overriden when using CLI :
# use -- to stop reading CLI configuration flags and resume reading arguments
npm <command> --param1 value1 --param2 value2 -- argument1
# default npm public registry (powered by CouchDB)
https://registry.npmjs.org
# registry configuration (defaults to public registry)
npm config list -l | grep -E "^registry\ =.*$"
# log in to the default registry, links specified scope to it
# adds login and token information to per-user config file
npm login --scope=@<scope>
# prints user authentified to configured registry
npm whoami
# log out from default registry, unlinks the specified scope and revokes the auth token
npm logout --scope=@<scope>
# lists all dependencies in the current project
npm ls --all
# forces the configuration to <global> then searches configured registry for packages containing <expr>
npm search --location global <expr>
# create a new package in scope <scope> ro changes the scope of an existing package (TBC)
npm init --scope=@<scope>
# simulate publishing of package.json folder <folder> to a public package in configured registry
npm publish --dry-run --access public --tag latest <folder>
# lists all tags for current project from configured registry
npm dist-tag ls
# removes tag <tag> from <pkg> in configured registry
npm dist-tag rm <pkg> <tag>
# searches configured registry for packages names matching <expr>
npm search <expr>
# prints details of package <pkg> from configured registry
npm view <pkg>
# forces the use of <registry> to simulate the installation of <pkg> in the current project
npm install --dry-run --registry <registry> <pkg>
# installs <pkg> and saves to package.json unless current node version is incompatible with package.json engine directive
npm install --save-prod --engine-strict <pkg>
# installs scoped package <pkg>
npm install @<scope>/<pkg>
# prints current/wanted/latest dependencies versions for current project
npm outdated
# updates all dependencies for current project (exercise caution)
npm update --save
# removes package from current project's node_modules and package.json (TBC does not remove node_modules/pkg directory itself)
npm uninstall --save <pkg>
# locally or remotely runs the command specified in <pkg>'s package.json "bin" field
# useful for running built-in CLI commands for modules installed as dependencies
npx <pkg> <arguments...>