Next.js overview
Next.js main features list
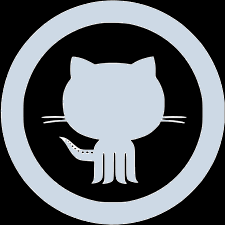
✔️ DX (fast refresh, linting, etc ...) vs production ready (bundling, minifying, etc ...)
✔️ Pages are pre-rendered at each request during development whatsoever to improve the DX
✔️ Exit Babel + Terser, enter SWC (Speedy Web Compiler - written in Rust)
✔️ JS + CSS automatically minified during the build process
✔️ Resolve dependency graph + treeshake + package
✔️ Optimize network traffic by associating each app entrypoint with its own bundle chunk
✔️ Building the app vs. delivering the build to end users
✔️ Build ouptut :
- Static HTML files
- JS code for rendering pages on the server
- JS code for making pages interactive on the client
- CSS files
✔️ I kind of know what it is ...
✔️ Next.js pre-renders every page by default :
- Data retrieval and transformation of react components to HTML happen server-side
- The resulting HTML page is sent to the client to display the UI
- This method eliminates render-blocking behavior by design, and results in better performance and SEO
- Pages can be pre-rendered using static site generation or server-side rendering
the result of pre-rendering shows when a page is opened while javascript is disabled ...
User-specific pages are private and irrelevant to SEO and thus should not be pre-rendered
✔️ Static Site Generation
- The page rendering only happens once, at build time
- The app is built into a static HTML website (no runtime required afterwards)
- Potential use cases are marketing pages, blog posts, e-commerce product listings, help and documentation ...
- Async fetching of data required for the static page can happen at build time using ```getStaticProps```
- ```getStaticProps``` runs on each request in dev mode
The question to ask is : "Can I pre-render this page ahead of a user's request?" If the answer is yes, then you should choose Static Generation.
✔️ Server-Side Rendering
- The page rendering happens on each request
- Each user agent request generates a new page in the server's memory
- The HTTP response contains HTML code, JSON data and JS code
- The user agent immediately displays the rendered HTML upon reception (progressive enhancement)
- Subsequently, the JS code runs and uses the JSON data to make the page interactive (hydration)
Server-Side Rendering has to be used if a page cannot be pre-rendered ahead of a user's request (For example if the page is designed to auto-updates or if the page content changes on every request)
In that case, you can use Server-side Rendering. It will be slower, but the pre-rendered page will always be up-to-date.
✔️ Client-Side Rendering
- No pre-rendering happens server-side, responses are sent immediately
- Data retrieval and react transformations happen client-side as usual
- Render-blocking behavior can happen
The last option is the traditional way of letting client-side JavaScript update the page when required
✔️ Resources optimization
- Next.js allows configuration of the rendering method on a page by page basis
✔️ Special feature : react server components
- These components are 100% rendered server-side and do not require client-side hydration at all
✔️CDNs store replicated static content and can deliver it to the user from the closest location
✔️Edges basically are CDNs that provide a runtime in which run small snippets of code can run
✔️...
✔️ Next.js can serve static assets from the top-level "public" directory
✔️ Files stored there can be referenced from the root of the application (similar to "pages")
✔️ The "next/image" component supports :
- On the fly resizing of images (do not deliver images whose size exceeds that of the viewport)
- Optimization of delivery format (for example, use ".webp" format if the browser supports it)
- Lazy loading of images (load only as they enter the viewport)
✔️ Next.js provides built-in components such as "next/head" that can be included in a page to add metadata
✔️ The "next/script" built-in component allow loading scripts hosted by third parties without impacting page performances