Docker services management
Scale and manage docker containers with services
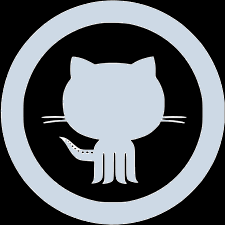
- This documentation is meant to be relevant primarily to the deployment of services in swarm mode by using
docker stack deploy
- All the commands mentioned here are swarm compatible unless specified and must be run on the swarm manager (ie. the node managing the swarm)
- Multiple services can be deployed on a swarm (whether using
docker service
ordocker stack
commands)
✔️ Docker swarm mode provides many additional capabilities for container-based applications :
- scaling
- networking
- securing
- maintaining
✔️ Swarms are not about dealing with individual containers :
- Instead, all swarm workloads are scheduled as services (scalable groups of containers based on the same image).
- Swarm mode adds and maintains service-wide networking features between containers.
- Swarm objects can and should be described in manifests called stack files and based on the compose file specification.
Note on services orchestration :
When services running on a swarm need to be orchestrated (ie. a given service will start successfully only if some other services are available), 2 strategies exist :
- Fine tune the restart policies of dependant service so that they initially fail, and then restart automatically once the services which are depended on are available
- Start the dependant services in replicated mode with zero replicas, and then run
docker service scale
to start the service containers once the services which are depended on are available
✔️ Initialize / dismantle a swarm
# initialize a docker swarm (the current node then become the swarm manager)
# optionally advertise the node interface, but this option is to be disregarded in most cases
docker swarm init --advertise-addr=eth0:2377
# make the current node join an existing swarm as a worker
# node must be capable to ping the swarm manager's ip address
docker swarm join --token <swarm_token> <swarm_manager_ip>:2377
# make the current node (worker or manager) leave the swarm
# if the manager leaves the swarm, said swarm is gone
docker swarm leave --force
✔️ Manage swarm-specific overlay networks
# create an overlay network on the current swarm for services to attach
# note : any compose application referencing this network will have to mention it as "external"
docker network create -o encrypted -d overlay <network_name>
# remove overlay network from swarm (no running services must remain)
docker network rm <network_name>
✔️ Setup / remove a service
# deploy a new service <service_name> based on <service_image> on the current swarm
# scale to <number_of_containers> containers
# publish service port <container_port> to node port <node_port>
# attach service to overlay network <network_name>
docker service create \
--name <service_name> \
--replicas=<number_of_containers> \
--publish target=<node_port>,published=<container_port> \
--network <network_name> \
<service_image>
# remove service <service_name> from swarm
# (stops all containers, unpublishes ports, detach from network)
docker service rm <service_name>
✔️ Setup / remove a stack
# creates a new stack called <stack_name> on current swarm using compose file <compose_file>
docker stack deploy -c <compose_file> <stack_name>
# removes stack <stack_name> from the swarm (stops all services and removes stack-specific overlay networks)
docker stack remove <stack_name>
✔️ Retrieve informations on running services
# list all services running on the current swarm
docker service ls
# list tasks (containers) for a specific service
docker service ps <service_name>
✔️ Update a running service
# scale service <service_name> to <new_number_of_containers> containers
docker service scale <service_name>=<new_number_of_containers>
# update service <service_name>'s base image to <new_service_image>
# all containers will gradually restart with the new image
docker service update --image <new_service_image> <service_name> --force