Docker networking overview
Fundamentals of docker networking
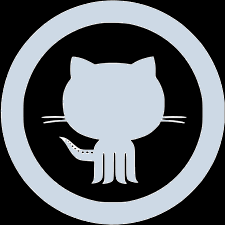
✔️ The docker networking subsystem allows containers to communicate with the outside using drivers :
- Bridge network :
- default network driver + a default
bridge
network is initialized by the daemon at startup (legacy, do not use) - a
bridge
network is managed by a single docker daemon, thus only containers running on a single host can connect to it - containers connected to the same
bridge
network can communicate with each other - containers connected to the same
bridge
network are isolated from the rest of the network - hostname resolution between containers can only happen on user defined
bridge
networks - all containers ports are published to each other if the containers are connected to the same
bridge
network
- default network driver + a default
# create a used defined bridge network
docker network create -d bridge <network>
# inspect a network (output connected containers names and addresses, network subnet, gateway etc...)
docker network inspect <network>
# creates a new container <name> from <image> in interactive mode, connects it to <network> and runs <command>
docker container run --network <network> --rm --name <name> -i -t <image> <command>
# connects a running container from an existing network
docker network connect <container> <network>
# disconnects a running container from an existing network
docker network disconnect <container> <network>
- Host network :
- the container's network stack is not isolated from the host (it shares the host's networking namespace)
- as opposed to the
bridge
driver, it won't create a new network interface on the host - from a networking point of view, it is the same level of isolation as if the container's command was running directly into the host
- you don't want to use the host driver on 2 or more containers running on the same host to avoid ports conflicts
- the ports publication options (-p) are ignored when using this network driver
# creates an interactive container from the busybox image using the "host" network driver
# typing ip addr show in the container will print the same network interfaces list as the host's
# any process listening to a port inside the container will effectively listen to the host's port
docker container run --network host --rm -i -t busybox /bin/sh
- Overlay network
- creates a distributed network among multiple hosts running docker daemons, which sits on top (overlays) the host-specific networks
- connected containers can communicate securely when encryption is enabled (
-o encrypted
). Docker handles packets routing to the relevant host/daemon/container - each time a daemon initializes or joins a swarm, two networks are created on the host running the daemon :
name | usage |
---|---|
ingress |
default overlay network, handles control and traffic related to swarm services |
docker_gwbridge |
built-in bridge network that connects deamon to other daemons of the swarm |
- the following ports have to be open to traffic to and from each host participating on an
overlay
network :
proto | number | usage |
---|---|---|
TCP |
2377 | cluster management communications |
TCP |
7946 | communication among nodes |
UDP |
7946 | communication among nodes |
UDP |
4789 |
overlay network traffic itself |
- notes :
- user-defined
overlay
networks can only be created ifingress
network is present - user-defined
overlay
networks can only be created on the swarm manager - docker services may then use the user-defined
overlay
network to deploy containers on a distributed network of nodes - docker services and stacks can only be created and deployed on the swarm manager
- user-defined
✔️ Initialize a docker swarm on host1
# the address advertising is optional (the command will return the join token)
docker swarm init --advertise-addr=<ipadresshost1>
✔️ Initialize host2 as a worker, join swarm
# initialize 'host2' as a soon-to-be worker
docker swarm init --advertise-addr=<ipadress>
# make 'host2' join the swarm managed by 'host1' using the join token
docker swarm join --token <token> --advertise-addr <ipadresshost2> <ipadresshost1>:2377
✔️ Create a user defined overlay
network on host1 (swarm manager)
# swarm management traffic encryption is enabled by default
# application data encryption is enabled (--opt encrypted)
# the above is not supported on windows nodes, such nodes won't be able to communicate
docker network create -o encrypted -d overlay <network>
✔️ Create a docker service named <name>
on host 1 (swarm manager) from <image>
using 5 replicas, publish service port 80 to node port 3000
# use service name <name>
# start 5 replicas (containers) from the image <image>
# publish service port 80 to node port 3000 (container port publishing is obviously irrelevant in swarm mode)
# set up service to use the user defined overlay network <network>
docker service create --name <name> --replicas=5 --publish target=80,published=3000 --network <network> <image>
✔️ For simplicity's sake, we will assume that overlay networks will always be used for service creation and stack deployments on swarms